Collisions + more gorbs
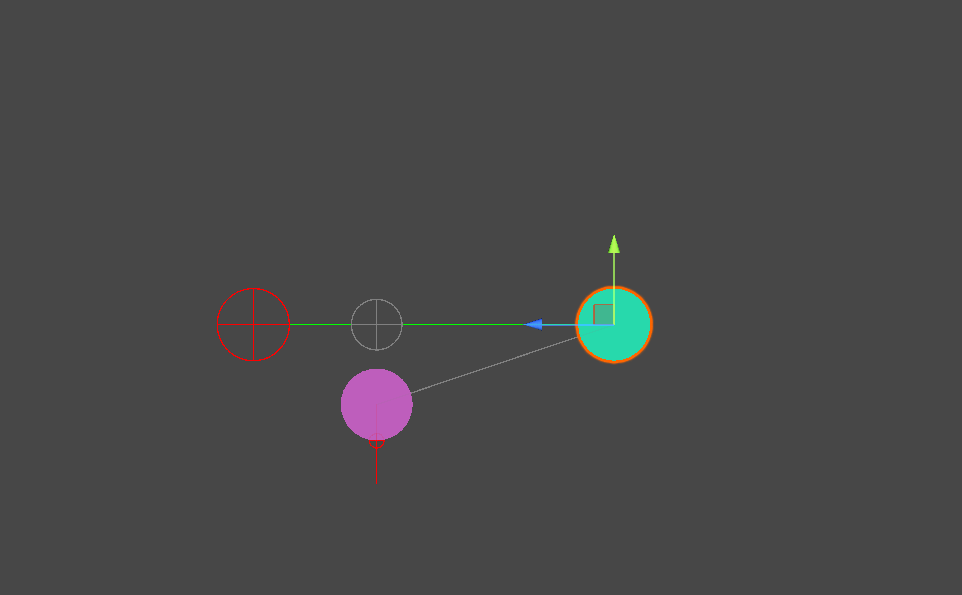
I've updated this a few times since I first posted it, but I haven't posted a devlog yet, and this was a fun feature to write about.
First, what I've done since the original build, off the top of my head:
- Added color sliders
- Added a black hole in the center of the universe
- Added live preview behind the setup menu
- Removed 2D mode (because it was boring)
- Changed default setup to gorbs orbiting attractors
- Added line/spiral preset
- Added binary system preset
- Fixed bug that made binary system unstable
- Didn't fix that bug for the gorbs, because it would get in the way of...
- Added (some) collision detection for gorbs
I have other things I need to be working on (hire me!) but this is a fun project to mess around with before/after normal day-to-day business. The two most obvious things to do are add more interesting setups and add more gorbs. I did a bit of the first, since it doesn't matter how much gravity you simulate if there's nothing to see. The limiting factor there is just the time it takes to make new UI to enable anyone to actually use the settings. I need to work on a better system for the UI, but that's a big boring task that has to wait until I have a weekend and some motivation to dedicate to it.
As for just adding more, that's the obvious thing to do, but I'm resisting the urge to go write compute shaders and all that. More interactions are more important, and I realized I could just throw some simple collision detection into the loop where the gorbs are calculating the gravitational force to each attractor. So I did that!
Collisions
I saw a video on youtube a while ago (can't find the link) of someone's GPU gravity simulation, and at the end they say "and I added collisions because they make everything better". Well I guess that wormed its way into my brain because for whatever reason I was looking at the gravity update loop and realized I could just do collisions in there too. I kind of looked at it like a "free" feature for that reason, but of course spent a few hours on it because nothing is free. The simplified pseudocode:
for (int j = 0; j < attractorCount; j++) { float force = (float)((g * mass) / sqdist); //...apply force to velocity // check for collision if(collision) { gorb[i].position = GetNewPositionAfterCollision(); goto next_gorb; //lol } } //later, apply velocity to position
An (un)important compromise is that because I'm avoiding a whole separate loop and quadtree etc search, I have to break out of the loop because, at least without doing a bunch of extra work, the velocity will be wrong for any further collisions. So that means if there are 5 attractors and it collides with the first one, the gravity of the next 4 won't be applied. This doesn't really matter because it's just for one frame and there are thousands of these things flying around so it isn't noticeable. Definitely not as noticeable as seeing gorbs bouncing off of attractors! Which really need better names.
The big attractors don't have collision detection right now, but that's not for any particular reason, they could easily use the same logic. It just feels like they should get something more interesting, or maybe they're fine with nothing.
Also, since this is in a nested loop, and I needed to break out of the enclosing loop, I used goto, which I vaguely know is considered bad from jokes I read before I even knew how to code. The stack overflow question about it and the MSDN docs apparently give this as the exact case you might want to use it. So I feel pretty good about having read that post for no reason at some point because having to refactor this probably would have made this take too long to finish in the time I had.
The actual math for detecting collisions comes from (as does all math) Inigo Quilez. But I am pretty happy that while implementing it I was able to find a couple of places to do some cute math tricks to avoid a square root here and there. I haven't profiled this in detail (the little optimizations are for fun more than performance) but it runs quick and I doubled the number of gorbs. Most of the frame time is taken up by the bloom effect that was a requirement of the original project.
The gif at the top is a little visualization I made to check my math (and figure out when things were wrong), that I might put on github or here, if it seems like it would be helpful to someone.
Files
GORBS 3D
Realistic gravitational chaos in 3D
Status | Prototype |
Author | loosegrid |
Genre | Simulation |
Tags | 3D, Abstract, Colorful, Experimental, Gravity, Mouse only, particles, Physics, Relaxing, Singleplayer |
Leave a comment
Log in with itch.io to leave a comment.